What is a class?
It is a blueprint to create multiple objects of the same type. Its like a template
How do you define a class?
class Personend
What is an instance?
A single object created from a class definition
How do you create a class instance?
employee = Person.new
How do you add a behavior to a class?
With a method, to access this behavior you would create an instance and call the method
What are attributes?
Values that persist inside an instance of a class, help with maintaining state
How do you store attributes?
Inside the instances, we use instance variables, because instance variables are persist throughout the instance
What is an Object?
It is a way to structure your code, it contains two things: data(attributes), logic(methods).
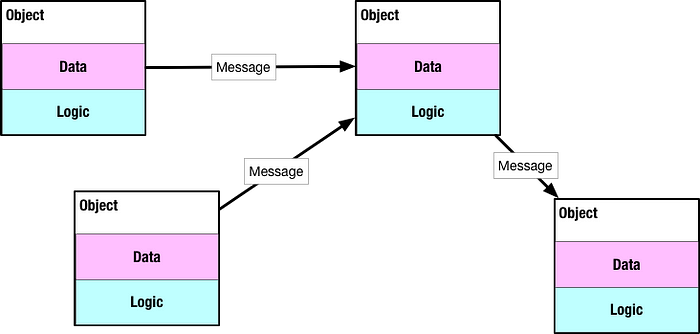
How does OOP work?
Encapsulation: Used to hide data from outside an object, data(attributes) and methods that work on are bundled together in one unit.
class Person
def initialize(name)
@name = name -> Instance variable
end
endemployee = Person.new("Tom")
employee
If you need to access the instance variable create a method and call it
class Person
def initialize(name)
@name = name -> Instance variable
end def name
@name -> can access data inside object
end
endemployee = Person.new("Tom")
employee.name => "Tom"
I do not like this pattern, I feel like a getter and setter are needed
class Person
def initialize(name) =>
@name = name
end
def get_name
@name
end
def set_name=(name)
@name = name
end
end
employee = Person.new
This pattern is not any better because the initialize method sets the initial value for an object, no need for set_name. Lets test out attr_accesor.
class Person
attr_accessor :name def initialize(name)
@name = name
end
def set_name=(name)
@name = name
end
def get_name
@name
end
endemployee = Person.new("Tom")
By using the attr_accessor you can set instance variables outside of the class.
class Person
attr_accessor :name
def initialize(name)
@name = name
end
end
So what is attr_accessor doing it is creating getter and setter method behind the scenes but how do you check.
class Person
attr_accessor :name
def initialize(name)
@name = name
end
endemployee = Person.new
puts employee.public_methods => name, name=
If you would like to learn more Andrew Livingston posted a great article about how attr_accessor works.
What is inheritance?
Permits code resusability by specifying common traits for different objects using parent child or is-a relationship.
What is the parent child relationship and how does it relate to superclass and subclass?
The superclass is the parent and the subclass is the child, the subclass can call a superclass method using the super keyword

Lets expand on the Person class when are been working with and see how inheritance works.
class Person
attr_accessor :name, :age def initialize(name, age)
@name = name
@age = age
end
def description
name + " is " + age.to_s + " years old"
end
endclass Child < Person
attr_accessor :hobbies def initialize(name, age, hobbies)
super(name, age)
@hobbies = hobbies
end
def get_hobbies
name + " hobbies are " + hobbies
end
endtom = Child.new("Tom", 25, "soccer")
tom.description
What is Duck Typing?
Duck types rely on common interfaces rather than inheritance
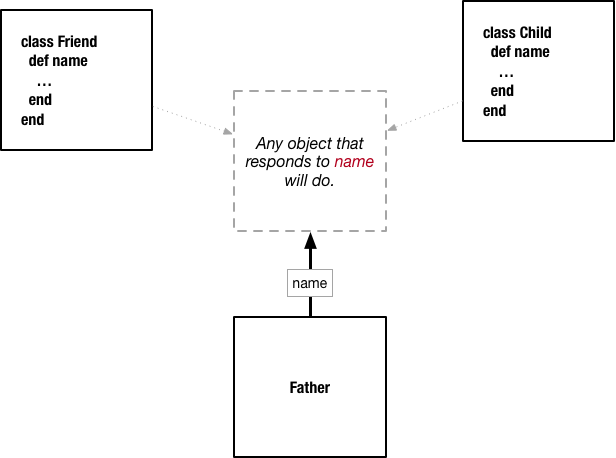
class Friend
def name
"Chris"
end
end
class Child
def name
"John"
end
end
class Father
def greeting(person)
"Hi #{person.name}"
end
end
Two completely different types are present but they respond to the same message